Loading Button

Android button widget that uses morph animation to transform into a circular progress bar.
It’s an easy way to make your application more responsive. You can customize the button in many ways to achieve the effect desired in your application.
TransformationLayout

With TransformationLayout you can transform one view into another through morph animation.
You can also use the morph effect for transitions between two activities or fragments. This is a very good-looking effect that will help your users better understand changes happening on the screen.
TransformationLayout library uses MaterialContainerTransform from the com.google.android.material:material package to achieve this result.
Fling Animation
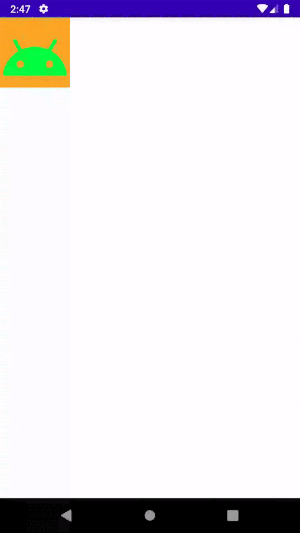
Use fling animation to simulate motion animation that takes into account the friction force.
You can customize friction and starting velocity. The friction force will be proportional to the animated object's velocity.
- Android’s official documentation for fling animation
- Use example
Animating Layout Changes

The android:animateLayoutChanges flag is a great and easy way to breathe life into your application.
You can use this flag on any ViewGroup. The Android framework will then automatically animate layout changes caused by any of the following actions on the parent view:
- Adding child view
- Removing child view
- Changing child view visibility
- Resizing child view
You might also customize the animation by modifying the LayoutTransition object.
Keep in mind that this API is quite old and limited so you won't be able to achieve every effect you need.
- Android’s official documentation for LayoutTransition
- Use example
Transitions API

The Transition framework makes it easy to animate various changes in your application’s UI by simply providing the starting layout and the ending layout.
You can describe what kind of animations you would like to use (fade in, fade out, translation) and the transition library will figure out how to animate from the starting layout to the ending layout.
You can use the transition framework to animate between activities or fragments. You can also animate simple layout changes.
Animate layout changes by calling:
TransitionManager.beginDelayedTransition(layout)
//apply changes to layout
You can also specify what kind of transition should be used for a particular view and configure animation order.
val transition = TransitionSet().apply {
ordering = TransitionSet.ORDERING_SEQUENTIAL
addTransition(ChangeColor())
addTransition(Explode().addTarget(secondImageView))
addTransition(Fade().addTarget(imageView).setStartDelay(1000))
}
TransitionManager.beginDelayedTransition(view as ViewGroup, transition)
Keep in mind that Transitions API has some limitations:
- Animations applied to SurfaceView or TextureView might not be displayed correctly.
- Classes extending AdapterView like ListView are incompatible with the Transitions framework.
- If you resize the widget with text then the text will not be animated. It will just pop to the final location.
Transitions API:
- Android’s official documentation for Transitions API
- Use example
Transitions API — Scenes
To animate between two layouts with the Transitions framework, you can use scenes API.
Steps needed to create the animation:
- Create two Scene objects — one for the starting layout and the second one for the ending layout.
- Create a Transition object. You can customize the type of animations and their order.
- Call TransitionManager.go(targetScene, transition).
Source: https://developer.android.com/training/transitions
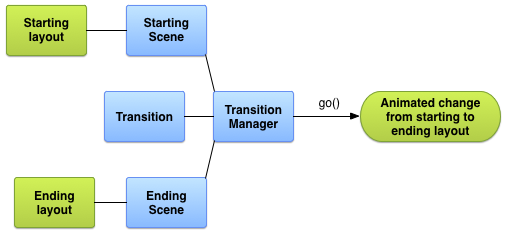

- Android’s official documentation for Scenes
- Use example
ConstraintSet

ConstraintSet lets you manipulate a set of constraints in ConstraintLayout.
You can combine it with Transition API to achieve complex animations easily!
- Android’s official documentation for ConstraintSet
- Use example
Activity Transition
Transitions API can also be used for activity transitions. You can define enter and exit transitions. You can also specify the shared elements between the two activities.
Thanks to this, the shared elements from one activity will seamlessly transition to their positions in the second activity.
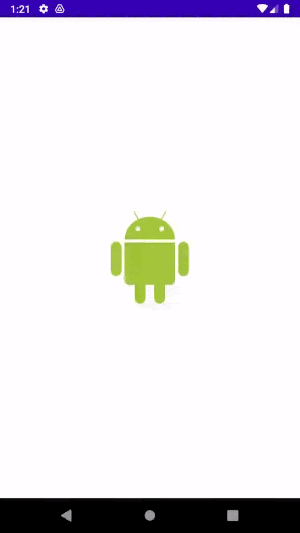
The gif shows the transition from one activity to another. The Android image is a shared element between those two activities.
Apart from specifying shared elements, you don’t have to do anything. Transitions framework will figure out animations on its own!
- Android’s official documentation for activity transitions
- Use example
MotionLayout — Basics
MotionLayout is a powerful layout that will let you create complex animations without a single line of code. It's fully declarative — you define transitions and interactions in XML.
It's a subclass of ConstraintLayout. Under the hood, MotionLayout uses the property animation framework and Transitions API.

In the gif below, you can see the debugging overlay. It will show you the paths of widgets and the animation frame rate.
You can enable it with <code> app:motionDebug="SHOW_ALL" <code> flag.

- Android’s official documentation for managing motion and widgets with MotionLayout
- Use example
MotionLayout — Key Frame Set

You can control how MotionLayout’s animation progresses thanks to KeyFrameSet.
It might be a little bit difficult to design complex animations with keyframes. Luckily you can use a cycle editor for that.
It's a tool designed by Google developers that will let you adjust key cycles in the GUI environment.
MotionLayout supports various keyframes:
- KeyPosition — specifies a view’s position during the motion sequence
- KeyAttribute — specifies a view’s attribute value during the motion sequence
- KeyCycle — adds oscillation to the animation. You can specify waveShape, waveOffset, and wavePeriod
- KeyTimeCycle — a keyframe that allows you to define a cycle driven by time instead of animation progress
Common keyframes attributes:
- framePosition defines the number of the frame (from 0 to 100)
- target defines which view should be modified
Android’s official documentation for MotionLayout. And our usage example.
Vector Drawables

Sometimes it's very useful to animate icons. For example, you might need to create a progress bar animation consisting of several images, or maybe you would like to create an animation where one icon morphs into another.
We have good news for you. You can delegate creating an animation to your designer! Shape Shifter will generate a drawable XML.
- Android’s official documentation for Vector Drawables
- Use examples
CoordinatorLayout

CoordinatorLayout can be used for many things, but you’ll most often use it in combination with AppBarLayout to achieve complex toolbar animations.
You can also create your own custom behaviors that will dictate how the CoordinatorLayout children interact with each other.
Take a look at ShrinkBehaviour as a reference. It demonstrates how to create a behavior that will shrink your FAB when the bottom panel slides in.
You can also create more complex behaviors — take a look at the SwipeToDismissBehaviour. This example illustrates the usage of ViewDragHelper, a powerful class that makes managing drag gestures much easier.
- Android’s official documentation for CoordinatorLayout
- Use example
CoordinatorLayout with BottomSheetBehavior

Another popular use case with CoordinatorLayout is a bottom sheet panel. Bottom sheet panel is a view that can be dragged by the user to reveal its content.
To achieve this effect, apply BottomSheetBehavior to a ViewGroup nested in CoordinatorLayout.
- Android’s official documentation for BottomSheetBahaviour
- Use example
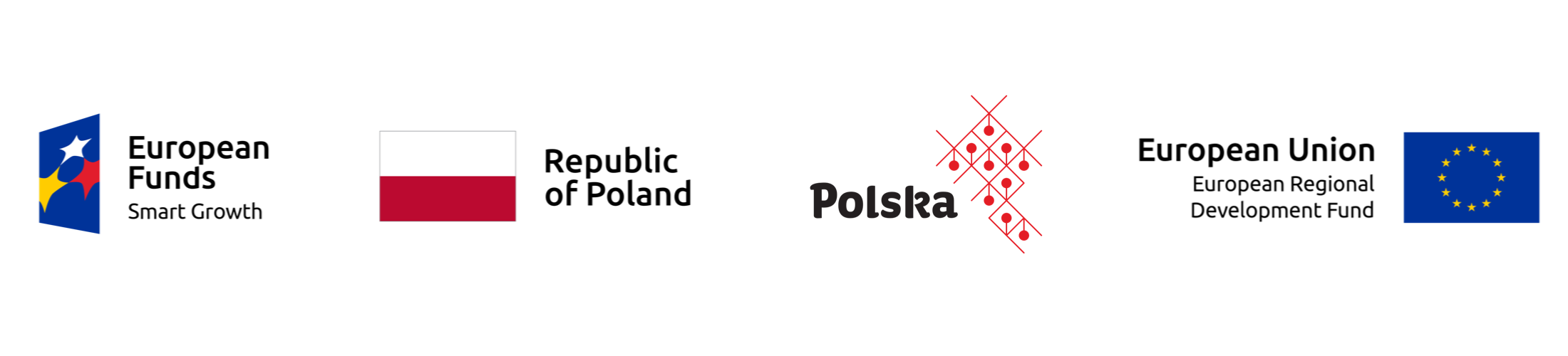
Related articles
Supporting companies in becoming category leaders. We deliver full-cycle solutions for businesses of all sizes.