What Is Code Refactoring, and Why Is It Important?
Code refactoring is the procedure that improves the software code without changing its existing functionality or the external application behavior. It involves cleaning the code for better clarity, readability, and maintainability. This process ultimately improves the application’s performance, scalability, and security. Refactored code is easier to debug and, most importantly, has fewer errors and bugs, lowering the likelihood of technical costs in the future.
Why Do We Need to Refactor Code?
To avoid code rot
A typical software development team consists of developers with different coding styles. During development, they keep updating the existing code. Sometimes, they can introduce correct but ugly code hacks as part of a quick solution, for example, in situations like a high-priority production incident or when trying to meet a quick deadline.
When there is no routine for refactoring such code, code rot can creep in. Code rot is when the code is getting cluttered and loses integrity. Examples of code rot include duplicated code, unnecessary dependencies, unused variables, or too many parameters. That’s why code refactoring should be introduced early to avoid bugs and errors in the application.
To save development effort and costs in the future
When the code is clear, existing and future developers can understand the code easily. This means you do not have to spend time explaining the code to your team or provide special training before adding new features to the application. Easily readable code saves a lot of your time and money and is the key to streamlined mobile app development.
To improve the application performance
Refactored code generally results in better performance of the product. If your application has third-party integrations, code refactoring helps keep well-maintained dependencies that don’t bloat the application. Code refactoring ensures that the desired levels of response times for application transactions and throughputs are met, which eventually improves customer experience.
When Should You Refactor?
During code review
Code reviewers might voice refactoring requests if they think portions of the code need it before merging with the codebase. Also, refactoring during a code review is most suitable if the code isn’t too big or the refactoring task is more straightforward. For example, writing a standard method won’t take much time if your code contains duplicate code.
Refactoring as a routine
You can also do refactoring proactively by allocating a specific period or day for this process as a good practice. For example, you can allocate a particular day a week to go through the developed code with the team and identify areas for refactoring, if any.
What Are the Techniques of Code Refactoring?
Red-Green-Refactor method
Red-Green-Refactoring is the most widely used code refactoring technique in an Agile environment where developers do Test Driven Development (TDD). In this method, the code undergoes three steps.
- Red — Write the failing test or stop and consider what you need to develop.
- Green — Write enough code to pass your tests.
- Refactor — Optimize and improve your code without introducing new functionalities.
Preparatory refactoring
Preparatory refactoring is another method that is a form of paying down the technical debts that have accumulated over time. As in Martin Fowler’s example, when you start developing a new feature, you find out that the code you are working on is not well structured and adding new features to that code is not easy. You can then restructure the code to make it easy to introduce the new feature into it. This refactoring before the actual development is called preparatory refactoring, where you prepare your code for better development.
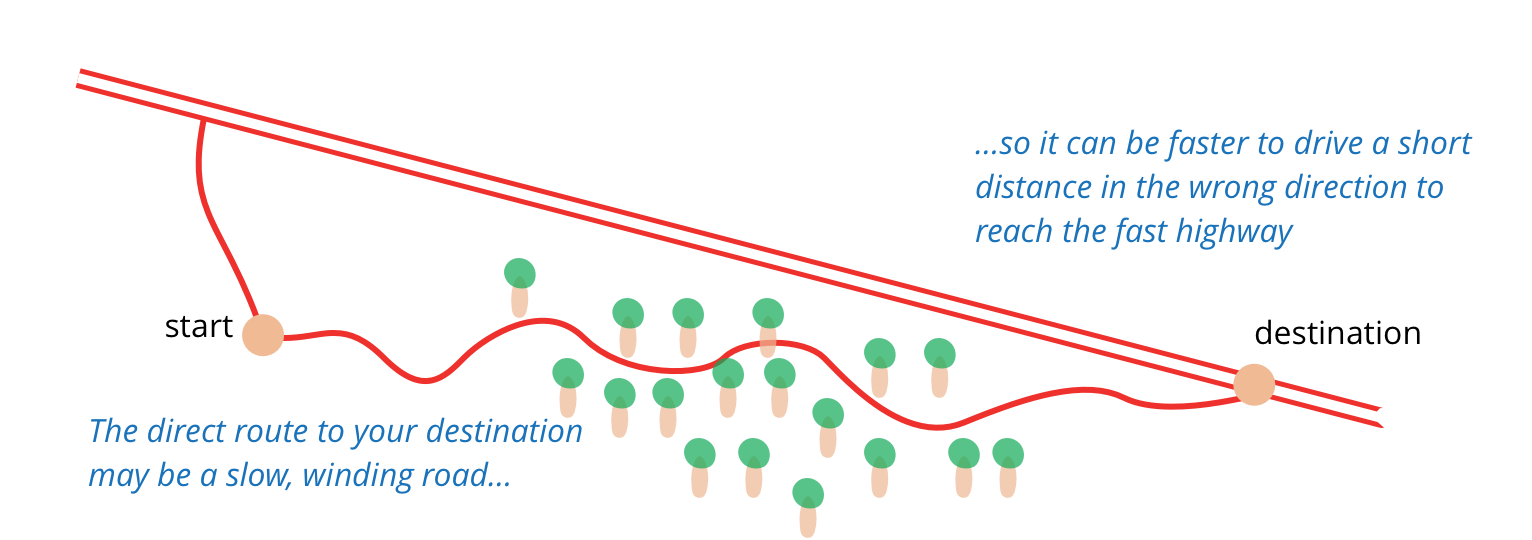
Refactoring by abstraction
This refactoring technique is usually best when you have a large chunk of code to refactor. The main goal of abstracting is decreasing the extent of duplication or redundancy in the code. In this method, developers build an abstraction layer with the code to be refactored. It mainly includes class inheritances, hierarchies, and interfaces. The most common example is the Pull-Up/Push-Down method.
- Pull-Up method: pulls or extracts the common code parts of the classes into a superclass to minimize code duplication.
- Push-Down method: push down the subclass-specific code parts from a superclass into the subclasses.
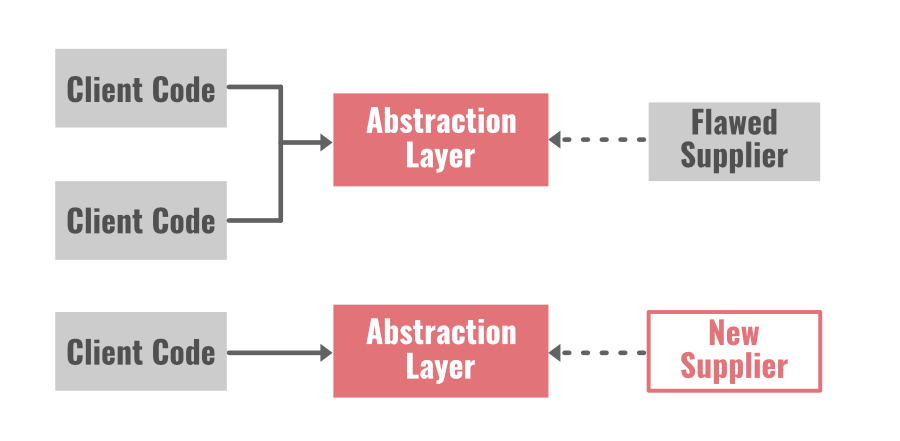
Moving features between objects
This method moves functionalities to new classes from old classes that have too much functionality. You can find out when it’s the right time to move between classes if you observe the class has too many functions defined in it or it doesn’t do much work. Then, you can move the features of that class into another class that has more space and is usable or remove it from the code.
Composing methods
Sometimes developers tend to write extended code that is too complex and difficult to read. To the rescue in situations like this comes the composing method for code refactoring. The extraction and inline are two of the techniques involved in this refactoring method.
Extraction — Breaking down the code into smaller parts and extracting the fragmentation. Then moving that fragmented code to separate methods.
Inline — Removing unnecessary methods to simplify the code as much as possible. To do that, search all calls to the considered method and replace those calls with the method body. The unnecessary method is now eligible for deletion.
Simplifying methods
Simplifying methods have two following techniques.
- Simplifying Conditional Expressions: Conditional statements in the code can become complicated over time. Simplify the conditional expressions using techniques like consolidating conditional expressions, decomposing conditionals, replacing conditional with polymorphism, etc.
- Simplifying Method Calls: This involves modifying the interactions between the classes. For example, updating the existing parameters and introducing new parameters, replacing the parameters with the method call, parameterizing method, etc.
User interface refactoring
In this technique, you can refactor the code by introducing simple changes to the UI. For example, apply a standard size to buttons and change the alignments of input fields so that the UI has a better visual appeal.
App Refactoring
Refactoring apps like iOS or Android improves their usability and portability for multiple app stores and platforms. It can be a part of an effort in importing old desktop applications to mobile applications. Let’s see in this section how we can do refactoring in IOS and Android application code.
How do I refactor in iOS?
Using the refactoring tool in Xcode
iOS developers often use Xcode, the integrated development environment (IDE) for building apps. Refactoring in this IDE is relatively straightforward because you can use the Xcode refactoring tool, which uses a refactoring engine to restructure your iOS application code. Using this tool, you can do both local and global refactoring. As shown in the following image, just select the code to refactor, right-click, and then select refactor.
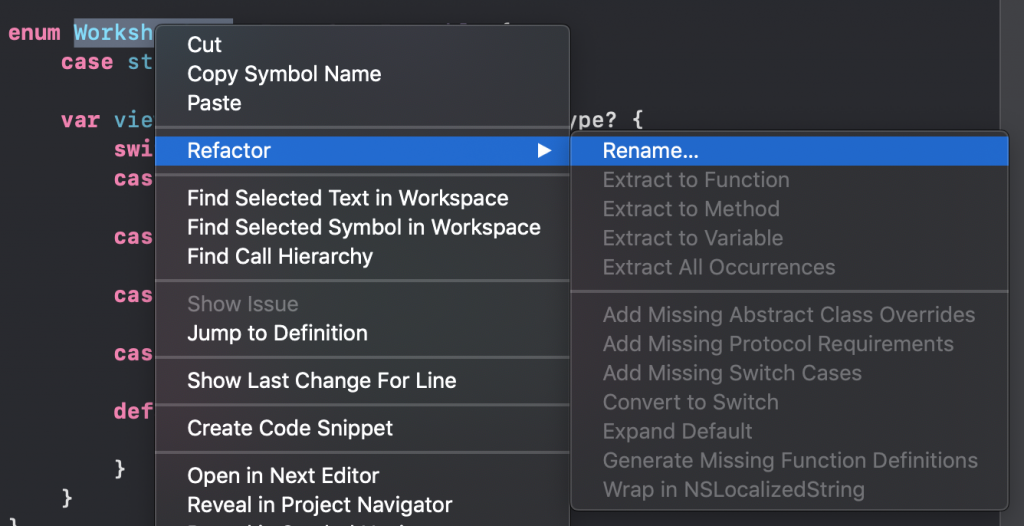
Extracting to Method — Extract to method function allows you to extract code pieces into different methods to make the code more readable.
Extract Variables and Extract All Occurrences — Extract multiple expressions to their variables. You can use the Extract to Variable method for a single expression, and for multiple occurrences, use the Extract All Occurrences method.
Edit all in scope — You can use this method to rename code inside a single file.
Create your refactoring method
Other than the refactoring tool, the open-source Xcode refactoring engine allows you to write your refactoring methods.
How Do I Refactor in Android?
Like in iOS development, refactoring in Android is also straightforward if you have the right tool. Android Studio, for example, provides a set of refactoring approaches making the refactoring process as painless as possible. Just highlight the code you want to refactor, right-click on it, and choose the refactor option from the menu. Read Jeroen Mols article for a detailed instruction on refactoring in Android Studio.
When Should You Not Refactor?
When the code lacks enough tests
If the code you're going to refactor doesn’t have a proper test, there is no way you can measure the performance gain after refactoring the code. For example, if a particular method isn’t in the application’s unit test suite or performance test suite, it’s difficult to check how refactoring has affected it. A better approach is to take the time necessary to complete the test coverage before stepping into refactoring.
When you’re on a tight release schedule
If you’re on a tight schedule for a critical release, reconsider your decision for refactoring unless you’re entirely sure that you can still meet the deadline. Once started, refactoring could take more time than you initially planned. You can always refactor in the next release.
How Do I Refactor Old Code?
Legacy code can be challenging to change because the patterns, libraries, classes, and many other types of code could be completely outdated and difficult to understand. Thus, legacy code refactoring needs to be done incrementally.
Here are a few tips based on typical code errors that show up in old codes:
- Split larger classes and interfaces into smaller classes and interfaces, creating new classes based on the function similarities
- Break lengthy functions or procedures into multiple methods that have a single or highly related responsibility. Formulate helper functions for code reusability where needed.
- Identify the usage of all the global variables. Avoid global variables by replacing them with function parameters.
- Follow TDD with unit tests for all the code functions making the code testable.
Code Refactoring Is a Sure-Fire Way to Keep the Code Clean and Well Maintained
Code refactoring is a crucial process that development teams need to embrace and practice continuously. Refactoring helps maintain well-standardized code, make it easier for developers who take over the project to work with it, and keep the performance of the app at the desired level.
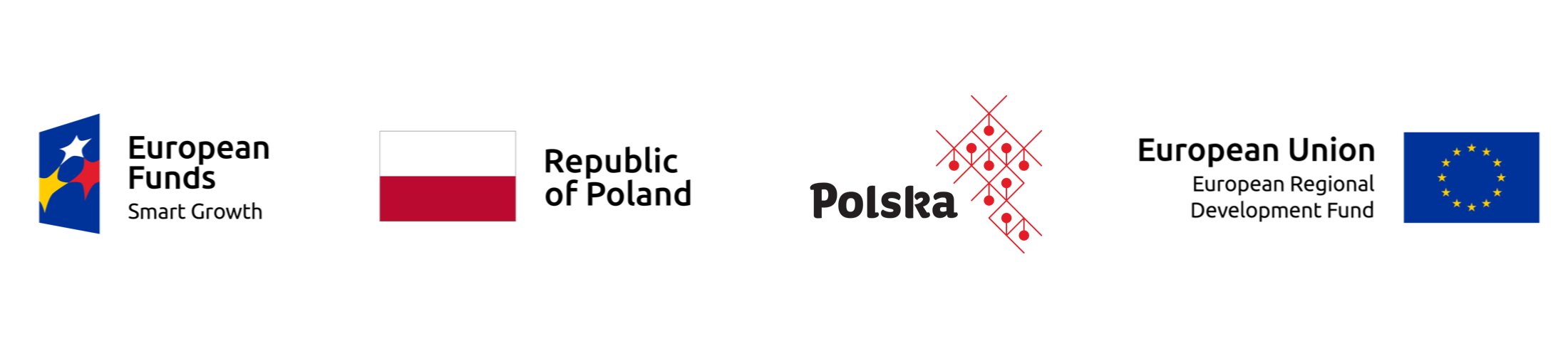
Related articles
Supporting companies in becoming category leaders. We deliver full-cycle solutions for businesses of all sizes.